As usual, I dove right in. Marked up all of my methods and member variables and was ready to go. But, ASDoc has quite a few arguments to customize the output and using the shell to run this every time I made a change got old real fast.
Now enter External Tools from the Eclipse framework. If you haven't used external tools in the past, basically it allows you to configure and run mind numbing tasks click and go style. After digging through the flex mailing lists for a bit, I came across this. Problem solved.
But.... I had several projects I wanted to document and I didn't want a million of these external tool configurations all over the place. Another nice feature of External Tools is variable substitution on workspace and project levels. So, here is how to add the external tool to run asdoc on the currently open project:
- Click Run-> External Tools -> Open External Tools Dialog
- Click on the New Configuration button
- Name it Generate ASDoc
- For location, enter the path to asdoc. On windows, the default is
C:\Program Files\Adobe\Flex Builder 3\sdks\3.0.0\bin\asdoc.exe
- In the working directory, enter
${project_loc}
- In the arguments input,
-source-path . -doc-sources ./com -window-title "${project_name}" -main-title "${project_name}"
- Click Apply
asdoc-oputput
that contains the fruit of our labor. If you open up index.html, it will look something like this: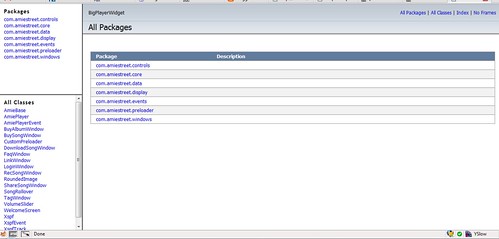
Pretty sexy, no?
About the Arguments
Here is a quick explanation of what the arguments in the above instructions do. You can view all of the available arguments here.
-source-path .
Sets the working path for asdoc. Why not use ${project_loc}
instead of setting the working directory? For some reason, external tools doesn't like spaces in its substitutions (even with proper escaping). Since the default workspace in flex builder contains spaces, this can be quite a pickle. Setting the working directory gets around this (thanks Jimmy!). -doc-sources ./com
Tells ASDoc to generate docs for everything inside ${project_loc}/com/
. If you are documenting a Flex Library Project, you can keep the code as is. Note: If you are trying to document a normal Flex Project (ie your code is in ${project_loc}/src/
), change the arguments appropriately.-window-title "${project_name}" -main-title "${project_name}"
Sets the HTML title and any other title references to the name of your project.